In Selenium tutorial series, this chapter, we are going to learn about the implicit and explicit waits feature present in Selenium WebDriver. These wait features play a vital role in test automation using Selenium WebDriver.
Why needs for Waits in Selenium?
In today’s world, most of the web applications are quite complex and make use of various asynchronous events such as AJAX Call, JavaScript procedure, etc. These events involve number of Web Elements which take different time to load by the browser. Therefore, in order to mitigate the situation where test completes without loading of the actual web element under test that results in “ElementNotVisibleException” exception, we use WebDriver wait feature in order to provide these web elements ample time to load before the test completes.
E.g., we have a web application which on a particular events loads a web element where the implicit wait time is set to 25 seconds and the explicit wait time is set to 15 seconds. In this application there is an expected condition i.e. explicit wait time to find a web element. If this web element is not located within the defined time frame then it will use implicit wait time of 25 seconds before throwing an exception known as “ElementNotVisibleException“
Let’s understand both implicit and explicit wait time with the help of following JAVA test script for Selenium WebDriver.
IMPLICIT WAIT
As explained above, the implicit wait method is the function that tells the WebDriver to wait for a particular time period in seconds to load a particular web element before throwing “ElementNotVisibleException” exception. It has the following syntax.
Syntax of Implicit Wait:
driverGC. Manage ().timeouts ().implicitlyWait (15, TimeUnit.SECONDS);
In the following example in Java test script, we are declaring an implicit wait that has a time frame of 15 seconds. It means that if the web element is not found on the current web page within this time frame, it will throw an “ElementNotVisibleException“.
package seleniumpackage;
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class ImplicitWaitDemo {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", ".//chromedriver.exe");
WebDriver driverGC = new ChromeDriver();
driverGC.manage().timeouts().implicitlyWait(15, TimeUnit.SECONDS);
String baseWebUrl = "https://www.linkedin.com/";
String expectedWebsiteTitle = "World’s Largest Professional Network | LinkedIn";
String actualWebsiteTitle = "";
driverGC.get(baseWebUrl);
/* get the actual value of the title*/
actualWebsiteTitle = driverGC.getTitle();
if (actualWebsiteTitle.contentEquals(expectedWebsiteTitle)){
System.out.println("Test Passed!");
} else {
System.out.println("Test Failed!");
}
driverGC.findElement(By.name("nosuchelement"));
driverGC.close();
System.exit(0);
}
}
Explanation of JAVA Code
In the above programme, we are doing the following stuffs.
- Firstly, we are instantiating the WebDriver for Chrome browser by using ‘ChromeDriver’ class.
- Next, we are loading the LinkedIn website URL to load the website home page.
- After this, we are calling an implicitlyWait method on line number 14 to allow the current web page to wait for 15 seconds before locating the given web element on the web page. This Implicit wait method accepts 2 parameters, the first parameter accepts the time as an integer value and the second parameter accepts the time measurement defined in the terms of MICROSECONDS, SECONDS, MINUTES, NANOSECONDS, MILISECOND, MICROSECONDS, HOURS, DAYS, etc.
driverGC.manage().timeouts().implicitlyWait(15, Timeunit.SECONDS);
- In this example, first we are fetching the title of the web page and comparing with the expected result that get passed here and the success message get displayed in the console.
- Secondly, we are trying to locate a web element that actually does not exits and purposely placed here, so that the current web page will wait for 15 seconds before print the exception “ElementNotVisibleException” as shown below in the console.
- Lastly, we are closing the instance of web driver and exiting the system.
Output Console for Implicit Wait WebDriver Method
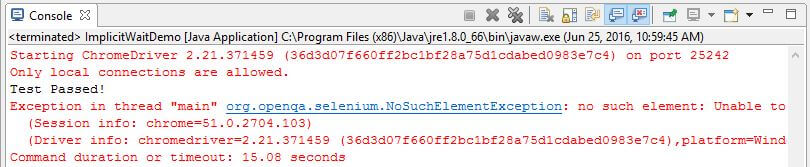
EXPLICIT WAIT
The explicit wait feature of the WebDriver API is used to inform the Web Driver to wait until a certain condition or ExpectedConditions is met within the given maximum time before throwing “ElementNotVisibleException” exception. It is a smart wait feature that can be applied only for specific web elements. Explicit wait are superior to implicit wait in the sense that an explicit wait waits for dynamically loaded Ajax elements.
Explicit Wait works with “ExpectedCondtions” class that can be configured to check the condition using Fluent Wait based on required frequency. Usually, we use Thread.sleep () method for introducing the wait time that is not recommendable when we have this explicit wait feature present in WebDriver API. It has the following syntax.
Syntax of Explicit Wait:
WebDriverWait driverWait = new WebDriverWait (driverGC, 25);
In the following example, we are defining a JAVA class “ExplicitWaitDemo” to demonstrate the use of explicit wait using WebDriver API.
package seleniumpackage;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class ExplicitWaitDemo {
public static void main(String[] args) {
System.setProperty("webdriver.chrome.driver", ".//chromedriver.exe");
WebDriver driverGC = new ChromeDriver();
WebDriverWait driverWait = new WebDriverWait(driverGC, 25);
String baseWebUrl = "https://www.linkedin.com/";
String expectedWebsiteTitle = "World’s Largest Professional Network | LinkedIn";
String actualWebsiteTitle = "";
driverGC.get(baseWebUrl);
/* get the actual value of the title*/
actualWebsiteTitle = driverGC.getTitle();
if (actualWebsiteTitle.contentEquals(expectedWebsiteTitle)){
System.out.println("Test Passed!");
} else {
System.out.println("Test Failed!");
}
WebElement webElement;
webElement = driverWait.until(ExpectedConditions.visibilityOfElementLocated(By.name("nosuchelement")));
webElement.click();
driverGC.close();
System.exit(0);
}
}
Explanation of Java Code
In the above programme, we are doing the following:
- Firstly, we are instantiating the WebDriver for Chrome browser by using ‘ChromeDriver’ class.
- Next, we are instantiating the ‘WebDriverWait’ class that accepts the instance of WebDriver and the wait time in seconds as shown below.
WebDriverWait driverWait = new WebDriverWait(driverGC, 25);
- Next, we are loading the LinkedIn website URL to load the website home page.
- After this, we are calling a ‘until’ method of the ‘WebDriverWait’ on line number 27 that accepts a single parameter as the “ExpectedConditions” class method ‘visibilityOfElementLocated’. This method expects the web element location by name, id, XPath, etc. In short, the wait time is defined by the “WebDriverWait” class which is expected on a particular web element with the “ExpectedConditions” class towards the visibility of that element on the web page. Syntax for the “ExpectedConditions” class is shown below.
WebElement webElement;
webElement = driverWait.until(ExpectedConditions.visibilityOfElementLocated(By.name("nosuchelement")));
webElement.click();
- In this example, first we are fetching the title of the web page and comparing with the expected result that get passed here and the success message get displayed in the console.
- Secondly, we are trying to locate a web element that actually does not exits and purposely placed here, so that the current web page will wait for 25 seconds before it gets times out and print the exception “ElementNotVisibleException” as shown below in the console.
- Lastly, we are closing the instance of web driver and exiting the system.
Output Console for Explicit Wait
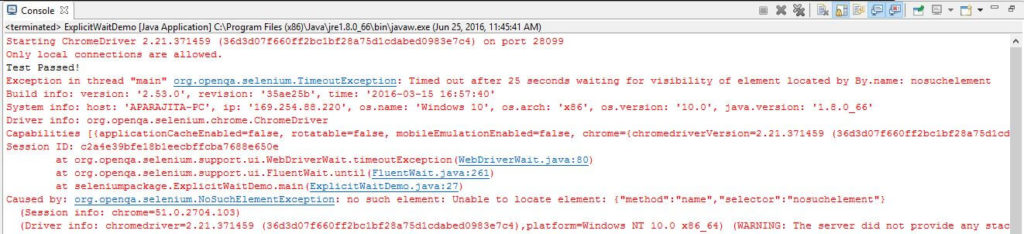
Conclusion
In this chapter, we have learned the use of implicit and explicit wait features in Selenium along with the test script example. In both cases, we have waited for a web element to load for the particular time duration in seconds. These features are very useful in implementation of the test automation for the applications that have AJAX calls and other asynchronous request model for dynamic loading of the web elements on the web page under test.
- What is Selenium Webdriver? – Selenium Training Series
- How to run your first Selenium WebDriver script – Selenium WebDriver Tutorial
- Why Selenium Server not required by Selenium WebDriver?
- Learn JUnit annotations used for your Selenium WebDriver automation
- How to use JUnit Annotations in Selenium WebDriver Automation Script
- Getting Started With Installation Of Selenium WebDriver – Learn Selenium
- How to Create Project in Eclipse IDE?
- Installation of TestNG in Eclipse – Selenium WebDriver Tutorial
- How To Access Forms In WebDriver
- How to handle Cookies in Selenium WebDriver